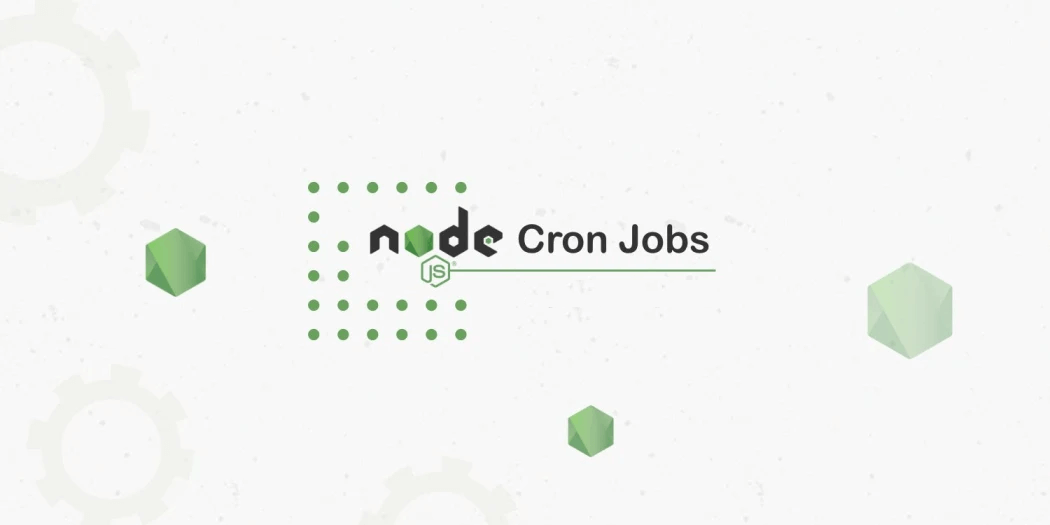
Where cron jobs come in?
Ever wanted to do specific things on your application server at certain times without having to physically run them yourself. Trust me there always comes a time in app development where you will want to automate certain aspects of our project. For example, run some scripts to import data into our system periodically from an outside database. This is where Cron jobs come in.
Table of Contents
What is cron?
Cron is a scheduler utility provided by Linux that allows you to schedule a job that can execute a command, run a script or perform a specific operation periodically.
How to set up a cron job in Node JS?
Cron jobs in Node.js can be set up using an external module known as node-cron. This module is based on GNU crontab.
1. Prerequisites
Node installed on your machine
NPM installed on your machine
Basic Knowledge of JavaScript
Create Node Application into your system
Install Node packages like express
Create Backend Server in index.js file using the express package
Then Listen to your Application with some random port e.g 4001
2. Installing node-cron
To install the module the following command is executed from the terminal
npm install node-cron --save
To use node-corn the module has to be imported in index.js file:
const cron = require('node-cron');
3. Setting up a Cron Job
The scheduling method is used to set up a cron job. This accepts 3 parameters :
Interval or the time at which the cron job is needed to run
The callback function contains the code to be executed
The third parameter is optional and passed as an object. The third parameter accepts 2 fields in an object form :
scheduled: accepts a boolean value. If set to false then the cron won't start automatically
timezone: accepts the timezone and will interpret the time set in the first parameter of schedule method accordingly
4. Basic Example of Cron Job
const cron = require('node-cron');
var task = cron.schedule('* * * * *', () => {
console.log('Printing this line every minute in the terminal');
});
5. Different Interval for Scheduling tasks
With node-cron, we can schedule tasks for different intervals. Let’s see how to schedule task using different intervals. In the example above, we created a simple Cron job, the parameters passed to the .schedule() function were * * * * * . These parameters have different meanings when used:
┌──────────────── second (optional) (Valid range 0-59)
| ┌────────────── minute (Valid range: 0-59)
| | ┌──────────── hour (valid range: 0-23)
| | | ┌────────── day of the month (Valid range: 1-31)
| | | | ┌──────── month (Valid range: 1-12)
| | | | | ┌────── day of the week (valid range: 0-7)
| | | | | |
| | | | | |
* * * * * *
6. Example of Cron Job with Timezone
The below code will print the line in the server every day when the time at "Asia/Mumbai" timezone is 08:20 Hours :
const cron = require('node-cron');
// cron won’t start automatically
var task = cron.schedule('20 08 * * *', () => {
console.log('Printing this line every day at 1750 Hours London Time.');
},
{
scheduled: false,
timezone: "Asia/Mumbai"
});
// start method is called to start the above defined cron job
task.start();
// stop method is called to stop already started cron job
task.stop();
// destroy method is called to stop and destroy already started cron job, after destroy you have to reinitialize the task as it is destroyed.
task.destroy();
7. Some Examples of setting up different time
If a cron job needs to be executed every second :
cron.schedule('* * * * * *', () => {
// code
});
If a cron job needs to be executed every minute :
cron.schedule('* * * * *', () => {
// code
});
If a cron job needs to be executed every hour :
cron.schedule('0 * * * *', () => {
// code
});
If a cron job needs to be executed every 10th date 8 hours and 30 minute :
cron.schedule('30 08 10 * *', () => {
// code
});
If a cron job needs to be executed in range of minutes between 10 to 20 each hour:
cron.schedule('10-20 * * * *', () => {
// code
});
If a cron job needs to be executed in 20th and 50th minute in each hour by passing multiple values:
cron.schedule('20,50 * * * *', () => {
// code
});
If a cron job needs to be executed every 10 mins:
cron.schedule('*/10 * * * *', () => {
// code
});
If a cron job needs to be executed at 08:10 AM on monday in February :
cron.schedule('10 08 * 2 1', () => {
// code
});
Conclusion
We saw how easy it is to set up a cron job in Nodejs and to use it in the Express server by using the cron-node library.
We saw different types of examples that help us in creating our cron job logic based on our requirements.
Here is a link that will be helpful in building node-cron schedule parameters : https://crontab.guru/#10_08_*_2_1

We are a team of expert developers, testers and business consultants who strive to deliver nothing but the best. Planning to build a completely secure and efficient Node app? 'Hire Node JS Developer'.
Third Rock Techkno is a leading IT services company. We are a top-ranked web, voice and mobile app development company with over 10 years of experience. Client success forms the core of our value system.
We have expertise in the latest technologies including angular, react native, iOs, Android and more. Third Rock Techkno has developed smart, scalable and innovative solutions for clients across a host of industries.
Our team of dedicated developers combine their knowledge and skills to develop and deliver web and mobile apps that boost business and increase output for our clients.