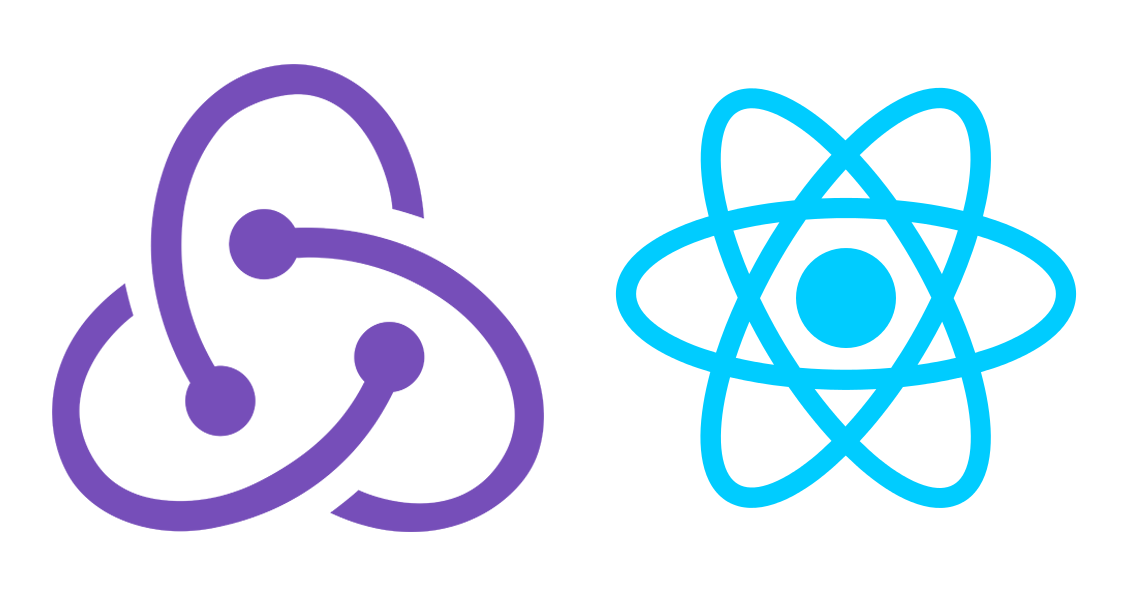
How to setup Redux using Redux Toolkit
Creating advanced Mobile apps includes not as it were UI building but moreover state management. One of the foremost widespread libraries for Redux.
What is Redux Toolkit?
Redux Toolkit is a global state management library. Redux Toolkit (RTK), once in the past known as Redux Starter Kit, gives a few alternatives for arranging the worldwide store and making activities and reducers more rapidly.
Create React Native app
npx react-native init ReduxToolKit
Install necessary libraries for Redux Toolkit
npm i react-redux @reduxjs/toolkit
Install pod for iOS
cd ios
pod install
Create a Redux Store file
Here we are going to create a store file with the name of src/redux/store/index.js Import the configureStore using @reduxjs/toolkit library. todoReducer is our reducer for storing created ToDo for the app. Initially we will create an empty Redux store and export it.
import { configureStore } from '@reduxjs/toolkit';
export const store = configureStore({
reducer: {},
});
Provide Redux store to React native App.
Here we will provide Redux to React native app by wrapping Provider to the components in the App.js file. Also import the Redux store which we had created and pass in the Provider as a props.
import React from 'react';
import {SafeAreaView, StatusBar, StyleSheet} from 'react-native';
import {Provider} from 'react-redux';
import {store} from './src/redux/store';
import ToDoList from './src/containers/Todo';
import {colors} from './src/themes';
const App = () => {
return (
<Provider store={store}>
<StatusBar barStyle="dark-content" />
<SafeAreaView style={styles.container}>
<ToDoList />
</SafeAreaView>
</Provider>
);
};
export default App;
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: colors.backColor,
},
});
Create a Todo Slice
Create a new file named src/redux/slice/todosSlice.js. In that file, import the createSlice API from Redux Toolkit. We will use the addTodo method as an action for this slice which will be helpful for storing data to the store.
import { createSlice } from '@reduxjs/toolkit'
let nextTodoId = 0;
const todoSlice = createSlice({
name: 'todo',
initialState: {
todos_list: [],
},
reducers: {
addTodo: (state, action) => {
state.todos_list = [...state.todos_list, {id: ++nextTodoId, task: action.payload}];
},
}
})
export const { addTodo } = todoSlice.actions
export default todoSlice.reducer
Add Slice Reducers to the store
Here we will import todo reducer for the todoSlice and add it to the store. With the use of todo field parameters inside reducers, we tell store to use todoSlice and handle all updates to the state.
import { configureStore } from '@reduxjs/toolkit';
import todoSlice from '../reducer/todoSlice'
export const store = configureStore({
reducer: {
todo:todoSlice
}
});
export type AppDispatch = typeof store.dispatch;
export type RootState = ReturnType<typeof store.getState>;
Use of Redux State, Actions and Components
Let’s create a TodoList where we can Add Todo with the help of TextInput and will display a list of Todos inside Flatlist.
In the TodoList component we will use useDispatch to use Redux actions in this component, With the help of Add button addTodo action will be triggered with todo text as a payload.
To fetch the stored record from the store we will use useSelector and display in the Flatlist.
import React, {useState, useRef} from 'react';
import {
SafeAreaView, KeyboardAvoidingView, FlatList, Text,
View, TextInput, TouchableOpacity, Keyboard } from 'react-native';
import Task from '../../components/tasks';
import styles from './styles.js';
import {strings} from '../../themes';
import {useDispatch, useSelector} from 'react-redux';
import {addTodo} from '../../redux/reducer/todoSlice';
const ToDoList = () => {
const flatListRef = useRef();
const [task, setTask] = useState();
const dispatch = useDispatch();
const todo = useSelector(state => state.todo);
const addTask = () => {
if (task.trim().length > 0) {
Keyboard.dismiss();
dispatch(addTodo(task));
setTask('');
}
};
const renderItem = ({item, index}) => {
return (
<TouchableOpacity key={index}>
<Task data={item} keyvalue={index} />
</TouchableOpacity>
);
};
return (
<SafeAreaView style={styles.safeAreaContainer}>
<KeyboardAvoidingView
style={styles.container}
behavior={Platform.OS === 'ios' ? 'padding' : 'height'}
keyboardVerticalOffset={Platform.OS == 'android' ? -270 : 10}>
<View style={styles.tasksWrapper}>
<View style={styles.headerBar}>
<Text style={styles.sectionTitle}>{strings.todaysTasks}</Text>
</View>
</View>
<FlatList
ref={flatListRef}
style={styles.listView}
data={todo?.todos_list}
renderItem={renderItem}
keyExtractor={item => item.id}
onLayout={() => flatListRef?.current?.scrollToEnd({animated: true})}
/>
<View style={styles.writeTaskWrapper}>
<TextInput
style={styles.input}
placeholder={strings.writeTask}
value={task}
onChangeText={task => setTask(task)}
/>
<TouchableOpacity style={styles.addWrapper} onPress={() => addTask()}>
<Text style={styles.addText}>+</Text>
</TouchableOpacity>
</View>
</KeyboardAvoidingView>
</SafeAreaView>
);
};
export default ToDoList;
Run React native app for iOS & Android
npm start
npm run ios
npm run android