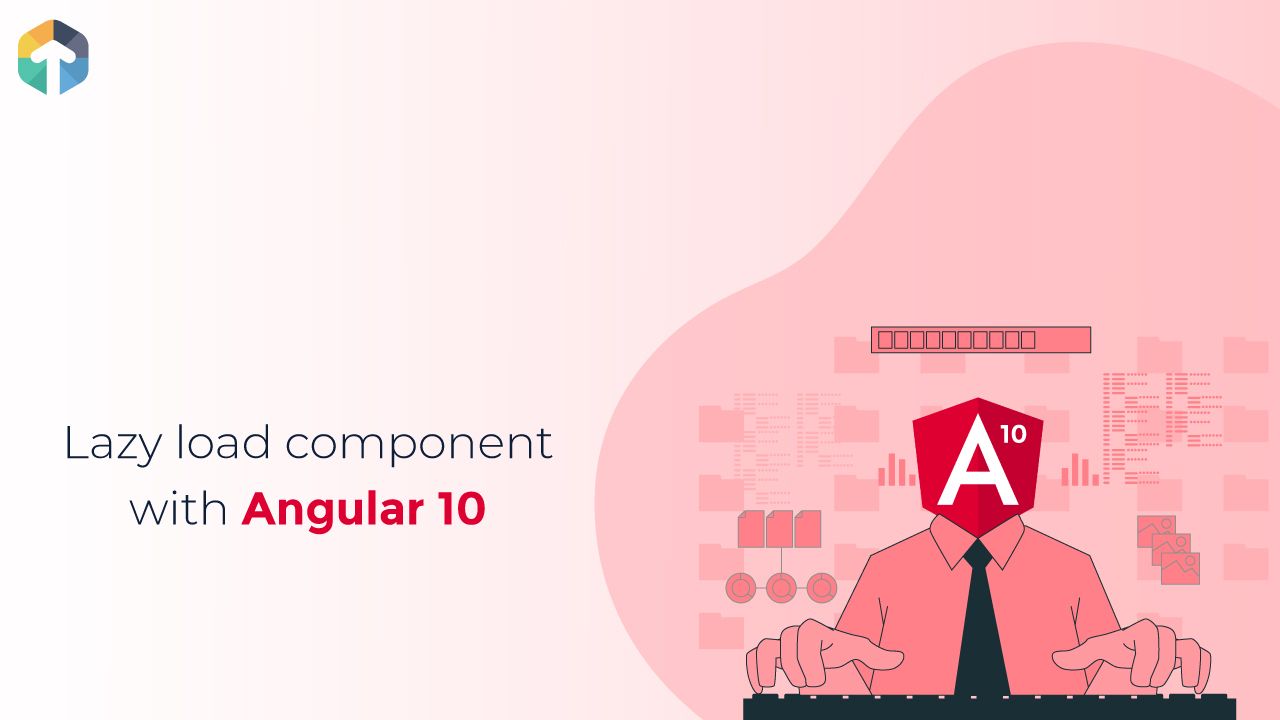
What is Lazy Loading?
Lazy loading (also called on-demand loading) is an optimisation technique for the online content, be it a website or a web app.
Table of Contents
Instead of loading the entire web page and rendering it to the user in one go as in bulk loading, the concept of lazy loading assists in loading only the required section and delays the remaining, until it is needed by the user.
By default, NgModules are eagerly loaded, which means that as soon as the app loads, so do all the NgModules, whether or not they are immediately necessary. For large apps with lots of routes, consider lazy loading—a design pattern that loads NgModules as needed. Lazy loading helps keep initial bundle sizes smaller, which in turn helps decrease load times.
There are two main steps to setting up a lazy-loaded feature module
Create the feature module with the CLI, using the
--routeflag.Configure the routes.
Prerequisites
Node version 12.0 installed on your machine.
Visual Studio Code
Angular CLI version 10.0
The latest version of Angular (version 10.0)
Create a feature module with routing
you’ll need a feature module with a component to route to. To make one, enter the following command in the terminal, where
customers
is the name of the feature module. The path for loading the
customersfeature modules is alsocustomersbecause it is specified with the--routeoption:ng generate module customers --route customers --module app.moduleThis creates a
customersfolder having the new lazy-loadable feature moduleCustomersModuledefined in thecustomers.module.tsfile and the routing moduleCustomersRoutingModuledefined in thecustomers-routing.module.tsfile.The command automatically declares the
CustomersComponentand importsCustomersRoutingModuleinside the new feature module.Because the new module is meant to be lazy-loaded, the command does NOT add a reference to the new feature module in the application's root module file,
app.module.ts.Instead, it adds the declared route,
customersto theroutesarray declared in the module provided as the--moduleoption.
ng add @nguniversal/express-engine --clientProject project-example
// app-routing.module.ts
const routes: Routes = [
{
path: 'customers',
loadChildren: () => import('./customers/customers.module')
.then(m => m.CustomersModule)
}
];
Add another feature module
Use the same command to create a second lazy-loaded feature module with routing, along with its stub component
ng generate module orders --route orders --module app.module
This creates a new folder called
orderscontaining theOrdersModuleandOrdersRoutingModule, along with the newOrdersComponentsource files.The
ordersroute, specified with the--routeoption, is added to theroutesarray inside theapp-routing.module.tsfile, using the lazy-loading syntax.
// app-routing.module.ts
const routes: Routes = [
{
path: 'customers',
loadChildren: () => import('./customers/customers.module')
.then(m => m.CustomersModule)
},
{
path: 'orders',
loadChildren: () => import('./orders/orders.module')
.then(m => m.OrdersModule)
}
];
Lazy Load Components
To explain the process of lazy loading a component, we are going to start with a simplified version of our
QuizComponent
which simplistically displays the question properties.We will then extend our component by adding Angular Material components. Last but not least, we react to output events of our lazy-loaded component.
So, for now, let’s lazy load a simplified version of the
QuizComponent
The first step is to create a container element. For this, we either use a real element like adivor we can use ang-container, which does not introduce an extra level of HTML.
<mat-toolbar>
<span>Third Rock Techkno</span>
</mat-toolbar>
<button class="btn btn-primary"
(click)="StartQuiz()">
Start Quiz
</button>
<ng-container #quizContainer class="quiz-container"></ng-container>
In our component, we then need to get a hold of the container. To do so, we use the
@ViewChildannotation and tell it that we want to read theViewContainerRef.
@ViewChild('quizContainer', {read : ViewContainerRef}) quizContainer : ViewContainerRef;
We got the container where we want to add our lazy-loaded component. Next, we need a
ComponentFactoryResolverand anInjectorwhich we can both get over dependency injection.
Ais a simple registry that maps Components to generatedclasses which can be used to create instances of components.
constructor(private cfr: ComponentFactoryResolver,
private injector: Injector,
private quizService: QuizService) {}
const QuizComponent = await import('./quiz/quiz.component');
const QuizFactoy = this.cfr.resolverComponentFactory(QuizComponent);
const instance = this.quizContainer.createComponent(QuizFactoy, null, this.injector);
instance.question = this.quizService.getNextQuestion();
Once the start button was clicked, we lazy load our component. If we open the network tab, we can see that the
quiz-component.js
chunk is lazy loaded. In the running application, the component is shown, and the Question is displayed.Advantages of Lazy loading:
On-demand loading reduces time consumption and memory usage thereby optimising content delivery. As only a fraction of the web page, which is required, is loaded first thus, the time taken is less and the loading of rest of the section is delayed which saves storage. All of this enhances the user’s experience as the requested content is fed in no time.
Unnecessary code execution is avoided.
Optimal usage of time and space resources makes it a cost-effective approach from the point of view of business persons. (website owners)
Disadvantages of Lazy loading
Firstly, the extra lines of code, to be added to the existing ones, to implement lazy load makes the code a bit complicated.
Secondly, lazy loading may affect the website’s ranking on search engines sometimes, due to improper indexing of the unloaded content.
Conclusion
Though there are certain pitfalls of lazy loading yet the big advantages, as optimal utilisation of the two major resources (time & space) and many more make us overlook its disadvantages.