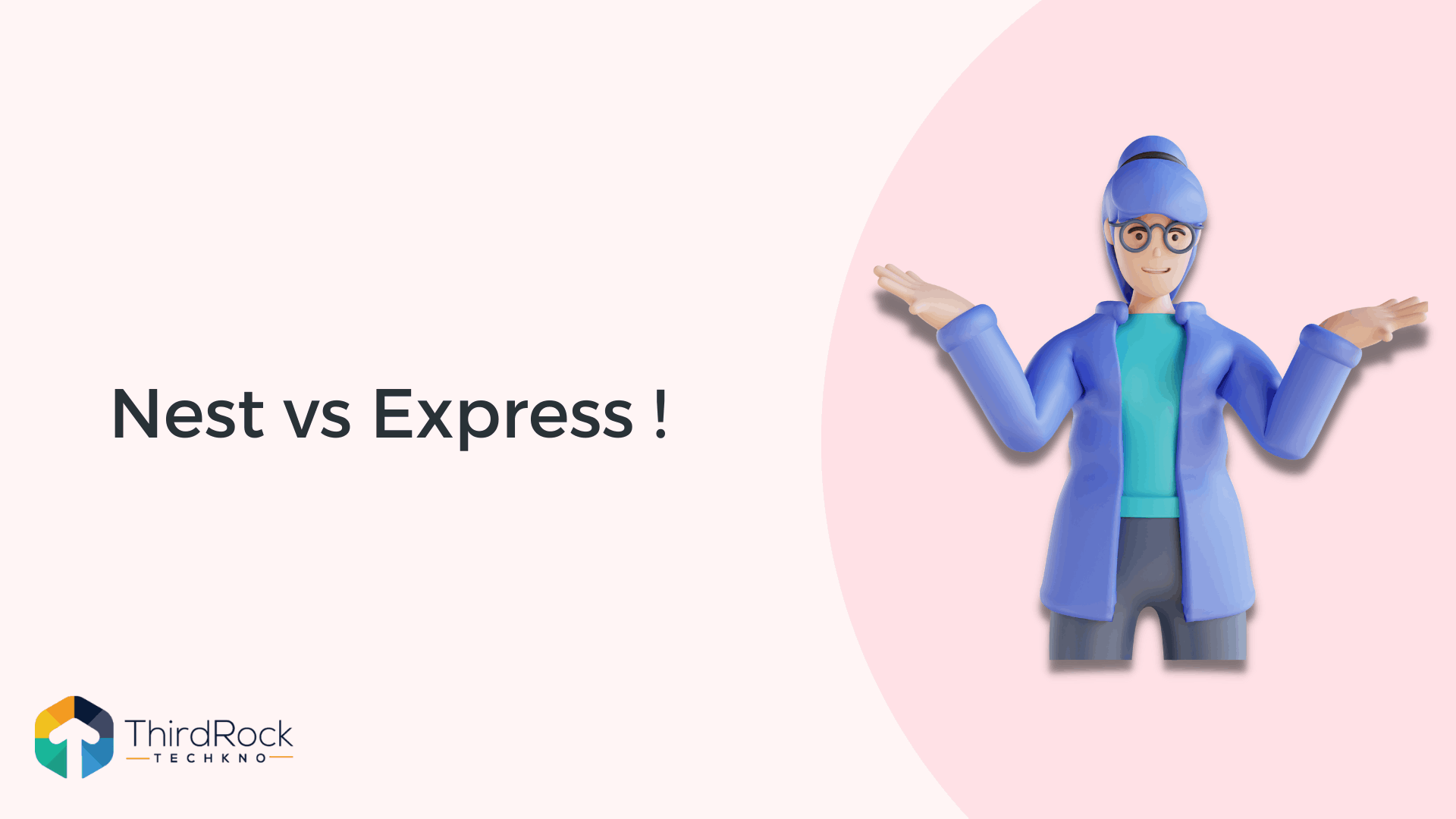
As a backend developer when you start planning to build a backend application using NodeJs and want to use some framework, Express.js is usually the first choice that comes to mind. Express has gained popularity in the last few years among developers.
Table of Contents
Nowadays Nest (NestJS) is another popular NodeJS framework that can help you to build large-scale applications with the support of typescript and some useful advanced design patterns like dependency injection, event driven development and MVC.
As both Nest and Express are used as frameworks for Node.js applications, there’s lots of discussion about which option is better for developers to start a new Node project. In this blog post, we’ll be comparing NestJS and ExpressJS to help developers make informed decisions.
So let’s start with the introduction of both frameworks and check key features.
1. Nest Introduction
Nest is a MIT-licensed open source framework for building efficient, scalable and enterprise-grade Node.js server-side applications. It uses progressive JavaScript, is built with and fully supports TypeScript but still enables developers to code in pure JavaScript (ES6, ES7, ES8).
Nest combines elements of OOP (Object Oriented Programming), FP (Functional Programming), and FRP (Functional Reactive Programming). In the background, Nest uses Express as the default http server and optionally can be configured to use Fastify as well.
Many superb libraries, helpers, and tools exist for NodeJS, but none of them effectively solve the main problem of - Architecture. Nest provides an out-of-the-box application architecture which allows developers and teams to create highly testable, scalable, loosely coupled, and easily maintainable applications.
2. Express Introduction
Express is a flexible Node.js web application framework that provides a set of features for web and mobile applications. Express provides many HTTP utility methods and middleware that can be helpful in creating REST APIs that are quick and easy. Many popular frameworks use Express like Feathers, LoopBack, Sails, Hydra-Express, Blueprint, graphql-yoga, NestJs etc.
3. Key features of Nest
TypeScript - A strongly typed language that is a superset of JavaScript, Nest is compatible with both TypeScript and pure JavaScript.
CLI - The Nest CLI is a command-line interface tool that helps you to initialise, develop, and maintain your Nest applications.
Organizing code: Nest supports two types of mode standard and mono-repo for code organization.
Modules: Lazy Loading modules that load things when necessary.
Dependency Injection: Contains a built-in DI container which makes applications modular and more efficient.
Exception filters, pipes, guards and interceptors available to handle and transform incoming requests and return response after applying your business or security logic.
Lifecycle Events - Nest provides lifecycle hooks that give visibility into key lifecycle events, and the ability to act (run registered code on your module, injectable or controller) when they occur.
Integration: Offers support for dozens of Nest-specific modules that help you easily integrate with common technologies and concepts like TypeORM, Mongoose, GraphQL, Logging, Validation, Caching, WebSockets, and much more
GraphQL - Nest support out of the box support for GraphQL it offers two ways of building GraphQL applications, the code first and the schema first methods using Apollo server and Mercurius.
Websockets - There are two WS platforms supported out-of-the-box socket.io and ws. You can choose the one that best suits your needs.
Serverless - Nest supports serverless development using @vendia/serverless-express and AWS-lambda modules to deploy your micro apps on lambda. There is also support for other platforms like azure and google cloud.
Testing: You can write test suites to automate testing of your application. It supports both unit and e2e testing with help of its module and it supports many testing framework integration of your choice.
Microservices: Nest supports several built-in transport layer implementations, called transporters, which are responsible for transmitting messages between different microservice instances.Provides easy to understand and detailed documentation & official courses to learn everything you need to master Nest and tackle modern backend applications at any scale.
4. Key features of Express.js
Flexible and Speedy - Express.js is very easy-to-use and flexible, and it’s faster than any other Node.js framework.
Express is a part of MEAN stack, a full stack JavaScript solution used in building fast, robust, and maintainable production web applications.
Easy to integrate with different template engines like Jade, Vash, EJS etc.
Powerful Routing System - The framework has the most powerful and robust routing system built out of the box that responds to a client request via a particular endpoint.
Middleware - Express is a routing and middleware web framework that has minimal functionality of its own: An Express application is essentially a series of middleware function calls.
Error Handling - Express catches and processes errors that occur both synchronously and asynchronously. It comes with a built-in error handler that takes care of any errors that might be encountered in the app.
Database integration - Adding the capability to connect databases to Express apps is just a matter of loading an appropriate Node.js driver for the database in your app.
5. Nest vs. Express
(a) Opinionated & unopinionated:
The main difference between both frameworks is that Nest is opinionated while ExpressJS is un-opinionated. An opinionated framework means that it has a proper style or way of doing things. It has a proper structure and is less diverse. An un-opinionated framework gives complete freedom to the developers and is very diverse but less structured.
The main difference between both frameworks is that Nest is opinionated while ExpressJS is un-opinionated. An opinionated framework means that it has a proper style or way of doing things. It has a proper structure and is less diverse. An un-opinionated framework gives complete freedom to the developers and is very diverse but less structured.
(b) Architecture:
Another difference between the two is their architecture. Nest provides ready-to-use components like controllers, providers, and modules with which you can easily implement UI and control logic and data. As ExpressJS doesn’t follow proper structure. As a result, your application might end up being inefficient and less optimized.
Another difference between the two is their architecture. Nest provides ready-to-use components like controllers, providers, and modules with which you can easily implement UI and control logic and data. As ExpressJS doesn’t follow proper structure. As a result, your application might end up being inefficient and less optimized.
(c) Typescript:
Nest is written in and supports TypeScript. As TypeScript follows status typing and includes a “type” feature, Nest is more reliable and suitable to develop large-scale applications. Express doesn’t support TypeScript by default but you can use some type modules and configure typescript to use with express.
Nest is written in and supports TypeScript. As TypeScript follows status typing and includes a “type” feature, Nest is more reliable and suitable to develop large-scale applications. Express doesn’t support TypeScript by default but you can use some type modules and configure typescript to use with express.
(d) Performance:
Performance is a key point while choosing a framework, Nest+Fastify gives great performance with structured code. Have a look at some benchmarks of Express, Nest with "@nestjs/platform-express", Fastify, Nest with "@nestjs/platform-fastify".
Ref. https://github.com/nestjs/nest/blob/master/benchmarks/all_output.txt
Performance is a key point while choosing a framework, Nest+Fastify gives great performance with structured code. Have a look at some benchmarks of Express, Nest with "@nestjs/platform-express", Fastify, Nest with "@nestjs/platform-fastify".
Ref. https://github.com/nestjs/nest/blob/master/benchmarks/all_output.txt
(e) Unit Testing:
Unit Testing is pretty easy and faster with Nest as its CLI includes a default testing environment configured in Jest. In ExpressJS, you need to configure the assertion library and test runner to write testing.
Unit Testing is pretty easy and faster with Nest as its CLI includes a default testing environment configured in Jest. In ExpressJS, you need to configure the assertion library and test runner to write testing.
(f) Core Components:
Nest’s core components are Modules, Controllers & Services while in Express we have Routes, Middleware and Templates.
Nest’s core components are Modules, Controllers & Services while in Express we have Routes, Middleware and Templates.
(g) Popularity:
ExpressJS is the older framework, it is the most popular and has a bigger user base. There are thousands of companies that use this framework. However, Nest is slowly gaining popularity as more and more people are shifting from Express to Nest. In fact, it ranks second among the top Node.js frameworks on GitHub with Express taking first place.
ExpressJS is the older framework, it is the most popular and has a bigger user base. There are thousands of companies that use this framework. However, Nest is slowly gaining popularity as more and more people are shifting from Express to Nest. In fact, it ranks second among the top Node.js frameworks on GitHub with Express taking first place.
6. Examples
(a). Bootstrap application
Nest
// File: src/main.ts
import { NestFactory } from '@nestjs/core';
import { ValidationPipe } from '@nestjs/common';
import { ApiCatsModule } from './api-cats.module';
async function bootstrap() {
const app = await NestFactory.create(ApiCatsModule);
const port = 3000;
console.log(`Cats app listening on port ${port}`);
await app.listen(port);
}
bootstrap();
// File: src/api-cats.module.ts
import { Module } from '@nestjs/common';
import { ApiCatsController } from './controllers/api-cats.controller';
@Module({
imports: [],
controllers: [ApiCatsController],
providers: [],
})
export class ApiCatsModule { }
// File: src/controllers/api-cats.controller.ts
import { Controller, Get } from '@nestjs/common';
@Controller('cats')
export class ApiCatsController {
@Get()
async getCats(): string[] {
return ['Cat 1', 'Cat 2', 'Cat 3'];
}
}
Express
const express = require('express');
const app = express();
const port = 3000;
app.get('/cats', (req, res) => {
res.json(['Cat 1', 'Cat 2', 'Cat 3']);
});
app.listen(port, () => {
console.log(`Cats app listening on port ${port}`);
});
(b) Routes
Nest
import { Controller, Post, Get } from '@nestjs/common';
@Controller('cats')
export class CatsController {
@Post()
create(): string {
return 'Cat created';
}
@Get()
findAll(): string {
return ['Cat 1', 'Cat 2', 'Cat 3'];
}
}
Express
app.post('/cats', (req, res) => {
res.send('Cat created');
});
app.get('/cats', (req, res) => {
res.json(['Cat 1', 'Cat 2', 'Cat 3']);
});
(c) Middleware
Nest
// File: src/common/middleware/logger.middleware.ts
import { Injectable, NestMiddleware } from '@nestjs/common';
import { Request, Response, NextFunction } from 'express';
@Injectable()
export class LoggerMiddleware implements NestMiddleware {
use(req: Request, res: Response, next: NextFunction) {
console.log('LOGGED');
next();
}
}
// File: src/app.module.ts
import { Module, NestModule, MiddlewareConsumer } from '@nestjs/common';
import { LoggerMiddleware } from './common/middleware/logger.middleware';
import { CatsModule } from './cats/cats.module';
@Module({
imports: [CatsModule],
})
export class AppModule implements NestModule {
configure(consumer: MiddlewareConsumer) {
// apply middleware
consumer
.apply(LoggerMiddleware)
.forRoutes('cats');
}
}
Express
// File: main.js
const express = require('express')
const app = express()
const myLogger = function (req, res, next) {
console.log('LOGGED');
next();
}
// apply middleware
app.use(myLogger);
app.get('/', (req, res) => {
res.send('Hello World!');
})
app.listen(3000);
Conclusion
Both Nest and Express are popular NodeJS frameworks that are easy to learn and get started. They both have their pros and cons. I personally suggest Nest for more manageable and structured code with microservice architecture that supports a variety of transport layers. Share your experience with both frameworks and don’t forget to share the framework for the next NodeJS project.
References: