Apollo Library has been used to Integrate GraphQl with React Web Application
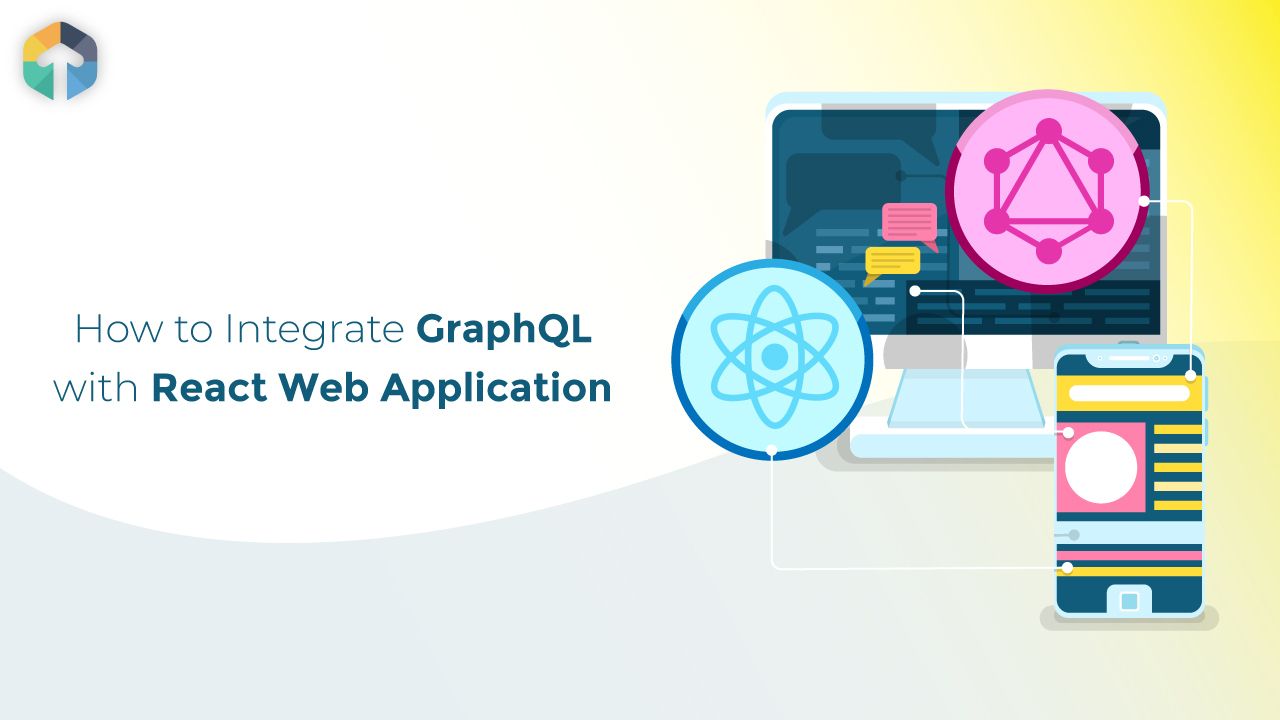
Table of Contents
How to Integrate GraphQL in React Web Application?
Have a Look to our Project structure :
How to use GraphQL Service in React Application :
#1 Create GraphQL endpoint URI backend server :
#2 Create Instance of ApolloClient :
#3 Connect ApolloClient to React App :
What is React Js?
React : A JavaScript library for building user interfaces.
React is an open-source JavaScript library for building user interfaces or UI components.
React can be used as a base in the development of single-page or mobile applications.
React allows us to create reusable UI components.
What is GraphQL?
GraphQL : A query language for your API
GraphQL provides a complete and understandable description of the data in your API, gives clients the power to ask for exactly what they need and nothing more, makes it easier to evolve APIs over time, and enables powerful developer tools.
GraphQL can be user in any application, web application or mobile app. In this tutorial you’ll learn how to use GraphQL in a React web application
How to Integrate GraphQL in React Web Application?
We need to create a new React Project and then install some dependencies of GraphQL libraries in it.
Prerequisites
- Basic Knowledge of JavaScript
- Node installed on your machine
- NPM installed on your machine
- Create New React Project : npx create-react-app react-graphql
- Go to Project Folder : cd react-graphql
- Run the project : npm start
- This open your application in browser : http://localhost:3000
Installing Dependencies
The next step is to install needed dependencies regarding GraphQL as bellow:
apollo-boost:
Package containing everything you need to set up Apollo Client
$ npm install apollo-boost
react-apollo:
Package is used for View layer integration for React
$ npm install react-apollo
graphql-tag:
Package is used to parse your GraphQL queries
$ npm install graphql-tag
graphql:
Package is also used to parses your GraphQL queries
$ npm install graphql
Have a Look to our Project structure :
In every React Application, important parts of the project are located in the src folder.
The main entry point of the React application can be found in index.js.
In this file we rendered out App component to the DOM element as root.
The implementation of App component can be found in App.js.
How to use GraphQL Service in React Application :
- Create GraphQL endpoint Backend server for URI
- Create Instance of ApolloClient
- Connect ApolloClient to React App
- Request Data by using Query component
#1 Create GraphQL endpoint URI backend server :
To create an Apollo endpoint back-end server for our React application
It has been created by certain ways:
- Create Node JS based GraphQL server by using Apollo Server
- Create Node JS based GraphQL server by using Express-GraphQL library
- Creating A GraphQL Server With Node JS And Express
- Use Apollo Launchpad to create a GraphQL server online
#2 Create Instance of ApolloClient :
To use GraphQL Service in React Application, we have to create instance of ApolloClient in App.js file, code is as bellow :
import ApolloClient from "apollo-boost";
const client = new ApolloClient({
uri: "https://vm8mjvrnv3.lp.gql.zone/graphql" //[GraphQL endpoint]
});
Imported ApolloClient from the apollo-boost library.
A new instance of ApolloClient is created and stored in client.
Instance has a configuration object with uri property.
#3 Connect ApolloClient to React App :
To make a connection between GraphQL endpoint and React App, we pass instance of ApolloClient to React App
Add bellow lines in App.js file :
import { ApolloProvider } from "react-apollo";
const App = () => (
<ApolloProvider client={client}>
<div>
<h2>Hello React + GraphQL app</h2>
</div>
</ApolloProvider>
);
The element is containing the template code which is used to render the component.
#4 Request Data by using Query component:
React Apollo Query library has been used to retrieve data from the GraphQL endpoint to your React component
The Query component used to embed the GraphQL query directly in the JSX code
Create a new file Courses.js in src folder and add bellow code to retrieve data:
import React from 'react';
import { Query } from "react-apollo";
import gql from "graphql-tag";
const Courses = () => (
<Query
query={gql`
{
allCourses {
id
title
author
description
topic
url
}
}
`}
>
{({ loading, error, data }) => {
if (loading) return <p>Loading...</p>;
if (error) return <p>Error :(</p>;
return data.allCourses.map(({ id, title, author, description, topic, url }) => (
<div key={id}>
<p>{`${title} by ${author}`}</p>
</div>
));
}}
</Query>
);
export default Courses;
Now Import Courses.js file in App.js with few changes :
import Courses from './Courses';
const App = () => (
<ApolloProvider client={client}>
<div>
<h2>List of Courses</h2>
</div>
<Courses />
</ApolloProvider>
);
Conclusion
- Here we Integrate GraphQL with React Web Application using Apollo library.
- We create pre-configured Apollo launchpad GraphQL Server, this might expired or deleted, Best option is to create your own Apollo launchpad GraphQL Server by using https://www.apollographql.com/blog/introducing-launchpad-the-graphql-server-demo-platform-cc4e7481fcba/
- Use can also create your Node, Express based GraphQL Server.
- For More details, refer https://developer.okta.com/blog/2018/10/11/build-simple-web-app-with-express-react-graphql

We are a team of expert developers, testers and business consultants who strive to deliver nothing but the best. Planning to build a completely secure and efficient React app? 'Hire ReactJS Developers'.