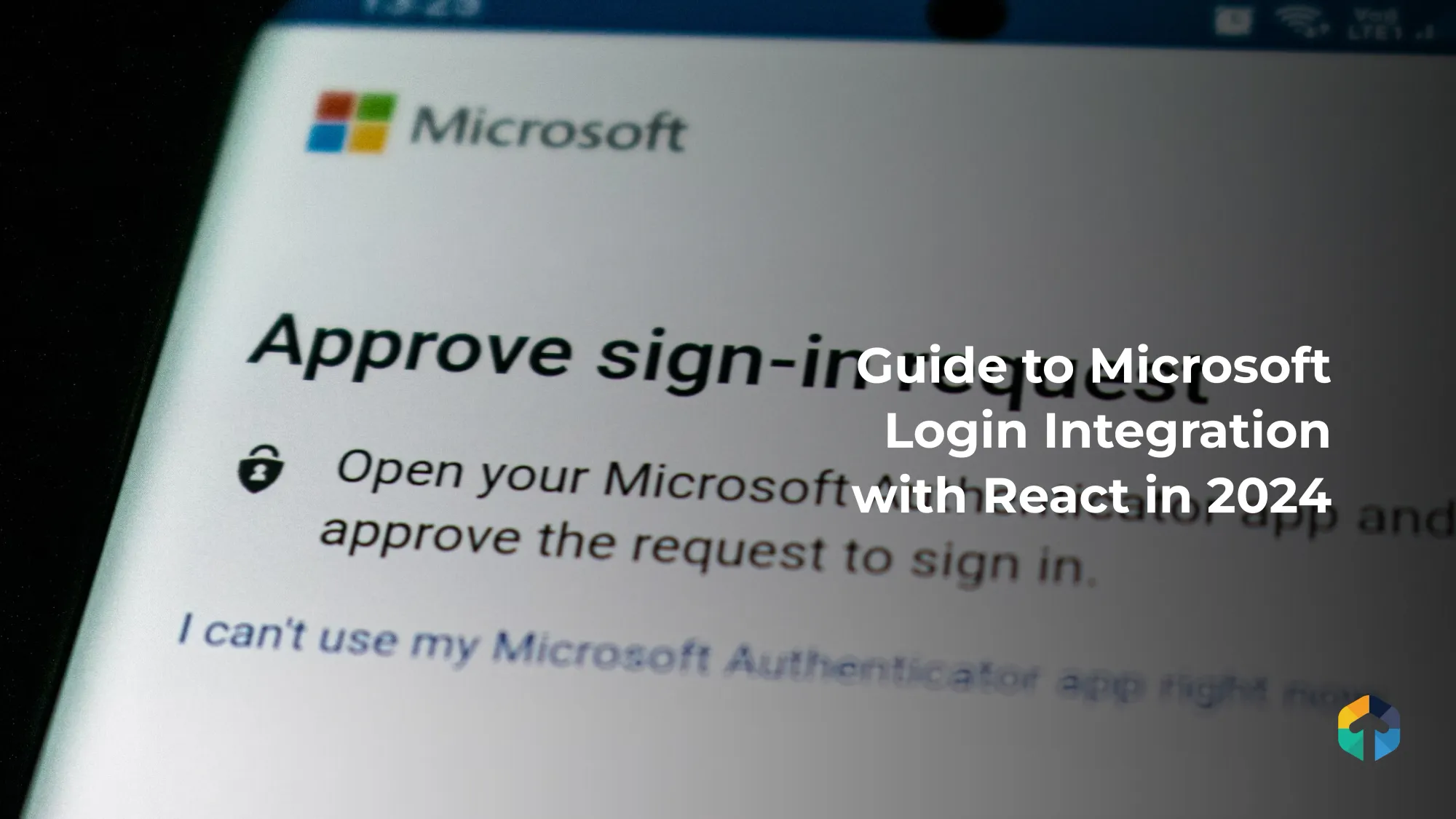
Let's talk about integrating Microsoft Login with React. Imagine your users logging in with the power of Microsoft it’s like giving them a VIP pass to your app! With Microsoft Login in React JS, your app can offer a seamless and secure login experience, leveraging the robust infrastructure of Microsoft.
Table of Contents
Plus, implementing Microsoft login in React is easier than you think. Whether you want to use the "sign in with Microsoft button react" or customize it to fit your app's style, the process is straightforward. Get ready to make your app shine with the credibility and convenience of integrating Microsoft login React.
Why Microsoft Login?
First things first, why should you even bother with Microsoft Login? Well, here are a few reasons
Security:- Microsofts got your back with top-notch security.
Convenience:- One less password for users to remeber
Credibility:- Integrating with Microsoft makes your app look super professional.
Sounds good. Let's get started!
Step 1:- Set Up Your Microsoft Application
Before diving into the code, you must set up an application on the Microsoft Azure portal. It’s like getting your backstage pass:
1. Create a New App:- Go to the Azure portal and create a new application. Give it a cool name.
2. Redirect URI:- Set the redirect URI to where you want users to land after they log in. Usually, it’s something like http://localhost:3000 during development.
1. Create a New App:- Go to the Azure portal and create a new application. Give it a cool name.
2. Redirect URI:- Set the redirect URI to where you want users to land after they log in. Usually, it’s something like http://localhost:3000 during development.
3. Client ID: Copy the Application (client) ID. This is like your secret handshake.
Step 2:- Install the MSAL Library
We use the Microsoft Authentication Library (MSAL) to make our lives easier. It’s like having a superpower cape for your app.
npm install @azure/msal-browser @azure/msal-react
Step 3:- Configure MSAL in Your React App
Now, let's sprinkle some magic into your React app:
Create a Configuration File: Create a file authConfig.js and paste in the following:
export const msalConfig = {
auth: {
clientId: 'YOUR_CLIENT_ID', // Replace with your client ID
authority: 'https://login.microsoftonline.com/common',
redirectUri: 'http://localhost:3000', // Replace with your redirect URI
}
};
2. Initialise MSAL: In your index.js file, add the MSAL provider
import React from 'react';
import ReactDOM from 'react-dom';
import { PublicClientApplication } from '@azure/msal-browser';
import { MsalProvider } from '@azure/msal-react';
import App from './App';
import { msalConfig } from './authConfig';
const msalInstance = new PublicClientApplication(msalConfig);
ReactDOM.render(
<MsalProvider instance={msalInstance}>
<App />
</MsalProvider>,
document.getElementById('root')
);
Time to add some bling to your app—a shiny "Sign in with Microsoft" button:
import React from 'react';
import { useMsal } from '@azure/msal-react';
const SignInButton = () => {
const { instance } = useMsal();
const handleLogin = () => {
instance.loginPopup().catch(e => {
console.error(e);
});
};
return (
<button onClick={handleLogin} style={{ padding: '10px', fontSize: '16px', background: '#0078D4', color: '#fff', border: 'none', borderRadius: '4px' }}>
Sign in with Microsoft
</button>
);
};
export default SignInButton;
Step 5: Display User Information
import React, { useState, useEffect } from 'react';
import { useMsal, useAccount } from '@azure/msal-react';
const ProfileContent = () => {
const { instance } = useMsal();
const account = useAccount();
const [profile, setProfile] = useState(null);
useEffect(() => {
if (account) {
instance.acquireTokenSilent({
scopes: ["User.Read"],
account: account
}).then(response => {
fetch('https://graph.microsoft.com/v1.0/me', {
headers: {
Authorization: `Bearer ${response.accessToken}`
}
})
.then(res => res.json())
.then(data => setProfile(data));
});
}
}, [account, instance]);
return (
<div>
{profile ? (
<div>
<h2>Welcome, {profile.displayName}!</h2>
<p>Email: {profile.mail}</p>
</div>
) : (
<p>Please log in to see your profile.</p>
)}
</div>
);
};
Export default ProfileContent;
Once users log in, let’s show them some love by displaying their info:
There you have it—your React app now has a swanky Microsoft login feature! With just a few steps, you’ve integrated Microsoft Login in React JS, making your app more secure, convenient, and professional. Now, go ahead and pat yourself on the back.
Let's look for some of the use cases that might help you. Shall we?
Use Cases for Microsoft Login Integration with React
Corporate Intranets
Integrating Microsoft Login into the company’s intranet makes it easy for employees to access important resources without remembering another password. They can log in with their existing Microsoft credentials and get straight to work, saving time and reducing frustration.Educational Platforms
Integrating Microsoft Login can streamline access to educational platforms for students and teachers. With a single sign-on, they can access coursework, assignments, and grades effortlessly. This integration can also improve collaboration through Microsoft Teams, making group projects a breeze.E-Commerce Platfrom
E-Commerce platforms can improve user experience by intergrating Microsoft Login. Shoppers can log in quickly with their Microsoft accounts, saving time and reducing cart abandonmnet rates. This seamless experience can increase user satisfaction and boost sales.Healthcare System
In healthcare, security and quick access to information are important. Integrating Microsoft Login in healthcare applications ensures that only authorized personnel can access sensitive patient data, maintaining privacy and compliance with regulations like HIPAA. Doctors and nurses can quickly log in and focus on saving lives.Financial Services
Integrating Microsoft Login adds an extra layer of security for financial services applications. Users can log in with their Microsoft accounts, leveraging Microsoft's advanced security features. This helps protect sensitive financial data and provides users with peace of mind.
Maybe Read:- How to Integrate GraphQL with React Web Application
Wrapping up!
There you have it! Microsoft robust infrastructure that you can offers a semaless secure login experience that users will appreciate. Not only does this integration improve security and convenience, but it also adds credibility to your app.
So, give yourself a pat on the back! You've just added a powerful feature to your React app that will make your users' lives easier and your app more appealing.
So, give yourself a pat on the back! You've just added a powerful feature to your React app that will make your users' lives easier and your app more appealing.
Happy coding!
FAQs
Configure your front end to include social login buttons and direct users to the provider's login page. Upon successful authentication, the provider will return user information, which you can use to log the user into your application.
2. How do I manage login in ReactJs?
To manage login in ReactJs, you can use libraries like React Router for routing and context API or Redux for state management. Set up a login form component to collect user credentials and send them to your backend server for authentication.
3. How do I add login authentication to React?
Adding login authentication to React involves creating a login form, handling form submission and managing the authentication state. Use a library like Axios to send login request to your backend server.

We are a team of expert developers, testers and business consultants who strive to deliver nothing but the best. Planning to build a completely secure and efficient React app? 'Hire ReactJS Developers'.