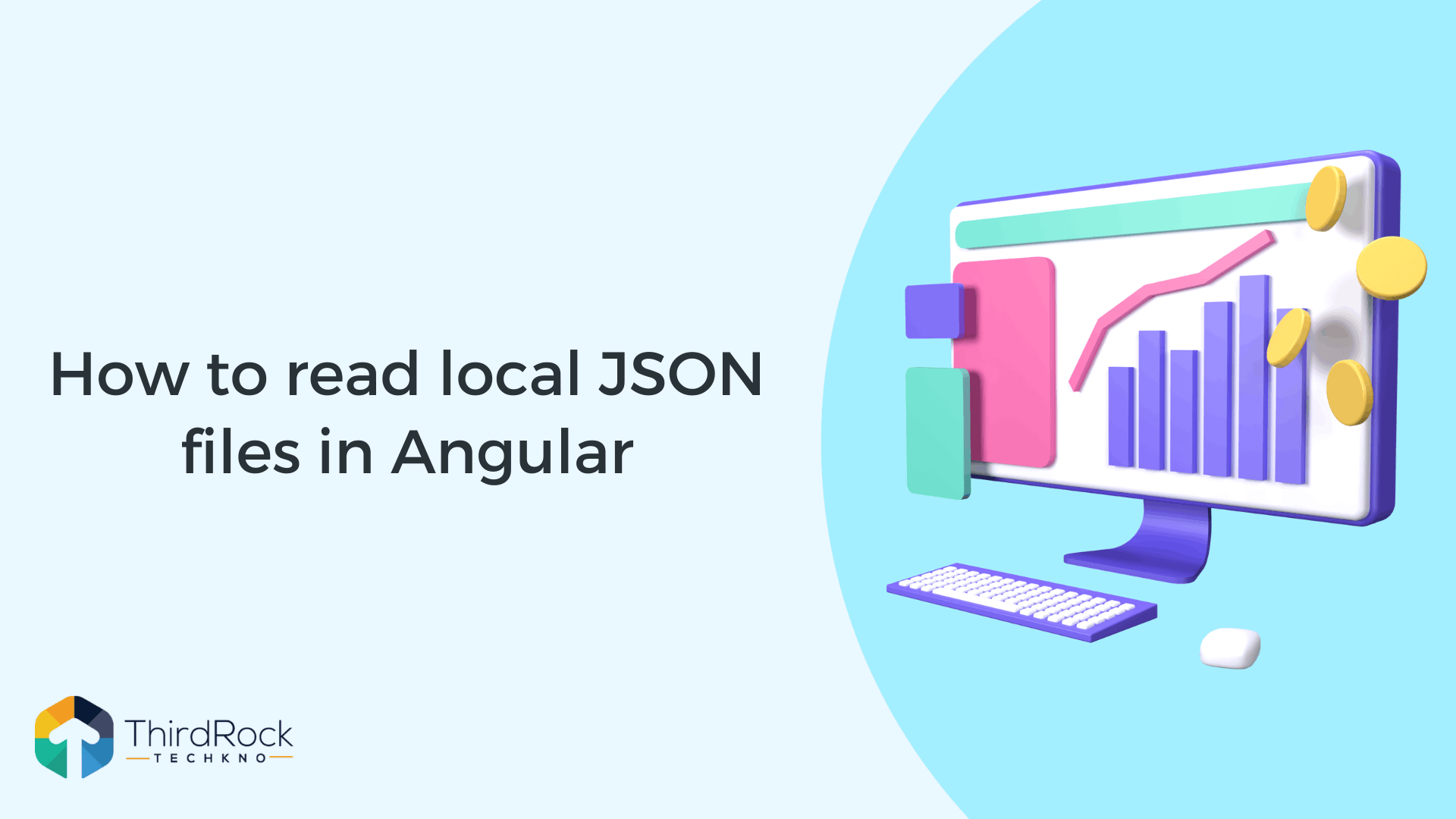
In this tutorial, I am going to create a sample application to show how to use local JSON files in an Angular application. There are different ways to read local JSON files in Angular. We’ll see different ways to read local JSON files in Angular, for example.
Table of Contents
It turns out there are at least a couple of ways to do it. I will mention the most common ones:
Using the
importstatementUsing Angular HttpClient
Step 1: Create a new Angular Project
Create an angular project using the CLI command:
ng new json-read-example
Step 2: Create a new JSON file under the assets folder
We’ll create dummy JSON data files that will contain a list of students.
[
{
"id": 1,
"name": "Luca",
"email": "luca@gmail.com",
"gender": "male"
},
{
"id": 2,
"name": "Lilly",
"email": "lilly@gmail.com",
"gender": "female"
},
{
"id": 3,
"name": "Anna",
"email": "anna@gmail.com",
"gender": "female"
},
{
"id": 4,
"name": "John",
"email": "john@gmail.com",
"gender": "male"
},
{
"id": 5,
"name": "Mary",
"email": "mary@gmail.com",
"gender": "female"
}
]
Step 3: Methods for Reading Local JSON Files
1. Using the import statement
One way to read a JSON file from the assets folder in Angular is to use the
import
statement in your component.import { Component, OnInit } from '@angular/core';
import * as studentData from '../assets/students.json';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent implements OnInit {
title = 'json-read-example';
data: any = studentData;
ngOnInit() {
console.log('Data', this.data);
}
}
You need to add
"resolveJsonModule": true
in the compilerOptions
of your tsconfig.json
the file that is at the root of your Angular application.{
"compileOnSave": false,
"compilerOptions": {
"baseUrl": "./",
"resolveJsonModule": true
},
"angularCompilerOptions": {
}
}
Also Check:- Angular Vs React For Web Development: How To Make The Right Choice?
2. Using Angular HttpClient
A second way to read a JSON in Angular from the assets folder in Angular is to use the HttpClient. Now, let’s see an example of it.
Import
HttpClientModule
in our root module. (app.module.ts
) like below:import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { HttpClientModule } from '@angular/common/http';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
In this case, we simply subscribe to the
Observable
generated by Angular HttpClient and log the data in the consoleThe HTTP protocol is utilized by the majority of front-end apps to connect with the server. When working with an application that uses Angular, we may make use of the HttpClient service available from the
@angular/common/http
package to read JSON files from an Angular application.import { Component, OnInit } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent implements OnInit {
title = 'json-read-example';
studentData:any;
url: string = '/assets/students.json';
constructor(private http: HttpClient) {}
ngOnInit() {
this.http.get(this.url).subscribe(res => {
this.studentData = res;
});
}
}
Display table view using the below file
<p>Read Local JSON file student data using typescript HttpClient</p>
<table id="student">
<tr>
<th>Name</th>
<th>Email</th>
</tr>
<tr *ngFor="let student of studentData">
<td>{{student.name}}</td>
<td>{{student.email}}</td>
</tr>
</table>
Some other way to read JSON file
use the fetch API
use angular jsonPipe
Use the fetch API
We use Javascript fetch API to retrieve our static JSON file, which is available in all browsers.
This is an example Angular 14 component that uses the Fetch API to read a JSON file:
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent implements OnInit {
title = 'json-read-example';
studentData:any;
url: string = '/assets/students.json';
constructor() {}
ngOnInit() {
fetch(this.url).then(res => res.json())
.then(json => {
this.studentData = json;
});
}
}
Use angular jsonPipe
This is good to know, even if it is not a way to read data from a JSON file.
Angular JSON pipe is mostly useful for debugging and this pipe creates a JSON representation of a data value.
The JSON pipe can be used as follows:
<p>{{ data | json}}</p>
Thank you everyone for reading the tutorial. I hope you enhanced your knowledge of Angular.
Conclusion
Reading and managing JSON files in Angular is an important skill for handling data effectively, especially for modern web applications. By using Angular JSON Assets, you can seamlessly integrate local JSON files into your project for dynamic content management.
Whether you're looking to read Angular from JSON files or want to better understand how to efficiently Angular read JSON files, the built-in features and services of Angular offers make it simple and efficient.
Wait! If you are looking for a partner who can help you scale your business. We at ThirdRockTechkno can help you grow your business. With over a decade of experience in custom software development, we will make sure your software meets your needs and expectations with on-time delivery. Contact us today!
FAQs
What are the common ways to read local JSON files in Angular?
There are several ways to read JSON files in Angular, but the most common approachis Using HTTP Client:- This is the most popular method. You can make a GET request to the local Angular JSON assets and retrieve the JSON file.Importing the JSON directly:- You can import a local JSON file in your Angular component or services by adding it to your assets folder and accessing it using require or TypeScript's import. These methods make sure smooth Angular load JSON file processes, helping with efficient data handling in applications.How to get specific data from JSON in Angular?
Once you have loaded your JSON file using HTTPClient or another method, you can retrieve specific data by parsing the response. For example, after fetching the data, you can use JavaScript methods.Is it possible to dynamically load a local JSON file at runtime in Angular?
Yes, Angular allows you to dynamically load local JSON files at runtime using the HTTP Client module. You can fetch JSON files from the assets directory or even an external API when needed. This flexibility makes Angular load JSON file a powerful feature.