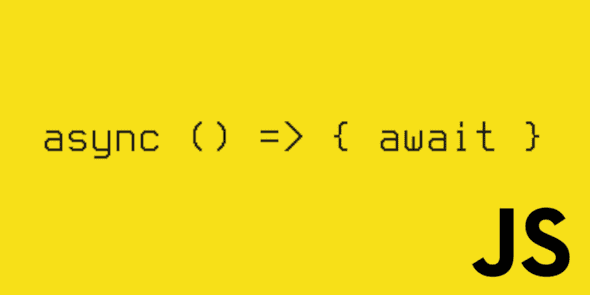
Asynchronous JavaScript has never been easy, earlier we use callbacks Then, we used promises. And now, we have asynchronous functions.
Asynchronous functions make it easier to write asynchronous JavaScript, but it comes with its own set of rules that makes life hard for beginners.
I will be sharing everything you need to know about Async functions so that you can run your project smoothly.
Asynchronous functions
Introduced in ES 2017 (ES8), asynchronous functions make working with promises much easier.
Here are some key features I would like to share it with you
- The most important aspect is that async functions work on top of promises. They are not fundamentally similar to the promise’s concept.
- An async function can be thought of as an alternate way of writing promise-based code.
- You can avoid chaining promise altogether using async/await.
- They allow asynchronous execution while maintaining a regular, synchronous feel.
An async function consists of two main keywords async and await. They keyword async is used to make a function asynchronous. The await keyword will ask the execution to wait until the defined task gets executed. It allows the use of await Keyword inside the functions with async keyword. Using await in any other way will cause a syntax error.
You can use it in a normal function declaration like,
async function functionName (arguments) {
// Do something asynchronous
}
Or you can also use it with arrow functions like,
const functionName = async (arguments) => {
// Do something asynchronous
}
But you should always make sure that
- The use of the async keyword happens at the beginning of the function declaration. In the case of arrow function, async is put after the “=” equal sign and before the “{}” parentheses.
We as a developer all know that functions are not the only way of writing an extensible code so we can also define it as an object as methods or in class declarations as below.
// As an object's method
const obj = {
async getName() {
return fetch('https://www.example.com');
}
}
// In a class
class Obj {
async getResource() {
return fetch('https://www.example.com');
}
}
You should always remember that async functions always return promises. It doesn’t matter what you return the returned value will always be a promise.
Here is an example:
const getOne = async _ => {
return 1
}
const promise = getOne()
console.log(promise) // Promise
Here is a sample code that I would ask you to give a try and see what output you get.
async function sampleFun(req, res){
let response = await request.get(‘https://www.thirdrocktechkno.com’);
if (response.err) { console.log('error');}
else { console.log('fetched response');
}
Here is my explanation.
If you see in the code above basically we ask JS engine running the code to wait for the execution of request.get() to be completed before moving to the next line for execution.
The request.get() function returns a promise for which the user/system will wait and thereafter execute the next block. Before async/await was introduced we needed to make sure that the functions are running in the desired sequence, that is one after the another, chain them one after the another or register callbacks.
I think one of the benefits of using the async/await is the code writing and understanding become easy as we see in the above example.
What you seen till now is the basic usage of the async/await. Now I shall share with you the more advanced use cases.
Asynchronous loop
Let’s just write an async function and await each task.
async function arrayProcess(array) {
array.forEach(async (item) => {
await func(item);
})
console.log('Done!');
}
Ideally, the system should wait for the execution of the forEach to be completed. But forEach will not wait until all items are finished. It will just execute the tasks and go to the next statement.
For our function to wait for the result we would have to use for await as shown in the example below. It will also ease the process of writing a complex loop and bring better readability:
async function arrayProcess(array) {
for await (const item of array) {
await getLogs(item);
}
console.log('Done!');
}
Note: The for await statement creates a loop iterating over async iterable objects as well as on sync iterable, including built-in String, Array, Array-like objects, Typed Array, Map, Set, and user-defined async/sync iterable.
Voila, now the code will handle each item one by one in series just as we wanted.
I would like to conclude this blog sharing that compared to using promise directly, not only can async/await makes the code more readable and understand. It also enables some interesting optimizations of Memory & performance too in JS Engine.
· · · ·Third Rock Techkno is a leading IT services company. We are a top-ranked web, voice and mobile app development company with over 10 years of experience. Client success forms the core of our value system.
We have expertise in the latest technologies including angular, react native, iOs, Android and more. Third Rock Techkno has developed smart, scalable and innovative solutions for clients across a host of industries.
Our team of dedicated developers combine their knowledge and skills to develop and deliver web and mobile apps that boost business and increase output for our clients.