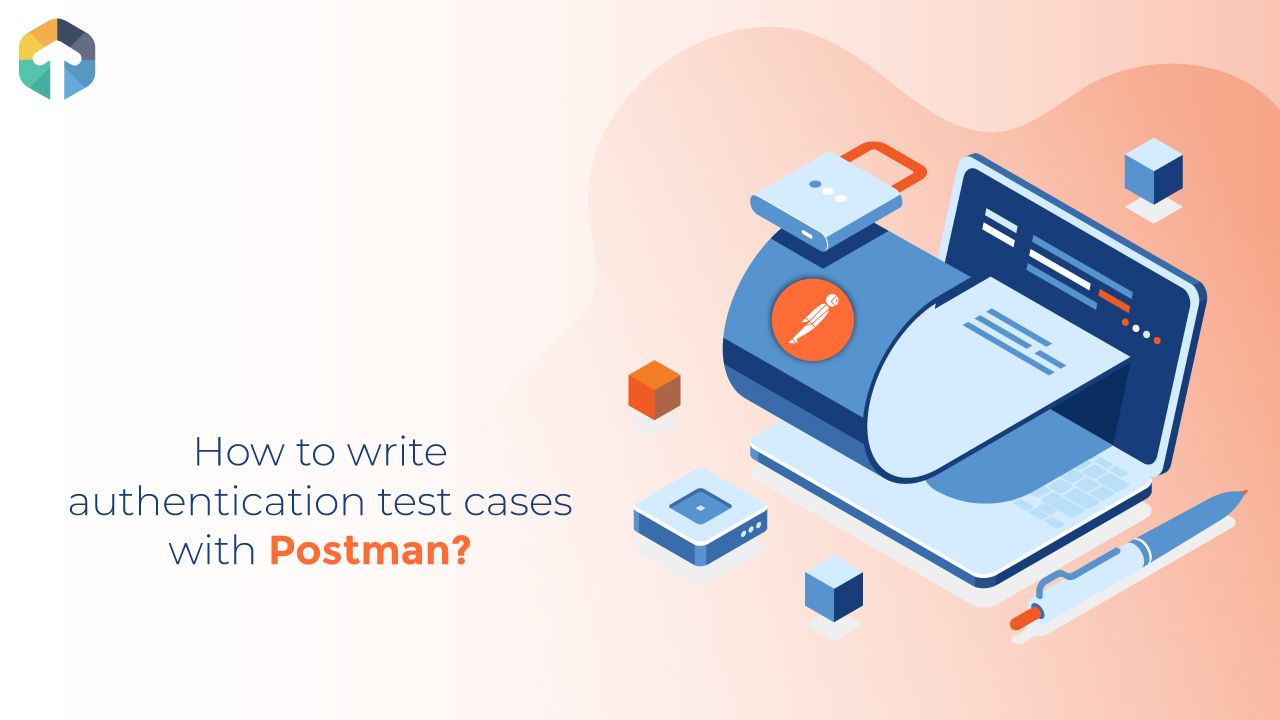
In Previous blog we have seen how we can use Postman CLI into our day to day for integration or sanity test suite.
Here in this blog I would like to share Various Types of Authentication provided by postman and how we can use in our API Testing.
Before we start with authentication with postman. I would like to share one more point like things we need to keep in the mind while API testing.
- Verify correct HTTP status code
- Verify response payload
- Verify response headers
- Verify response with JSON schema
Also, need to cover test scenario like:
- Testing API with valid input
- Testing API with invalid input
- All Positive and Negative Scenario including all optional parameters
There are various methods for Authentication provided by postman. Some of the few methods I am going to show here.
Basic Auth
How to set Basic Auth ?
- Navigate to the Authorization Tab and Select Basic Auth.
- Add API for Basic Auth with username and password.
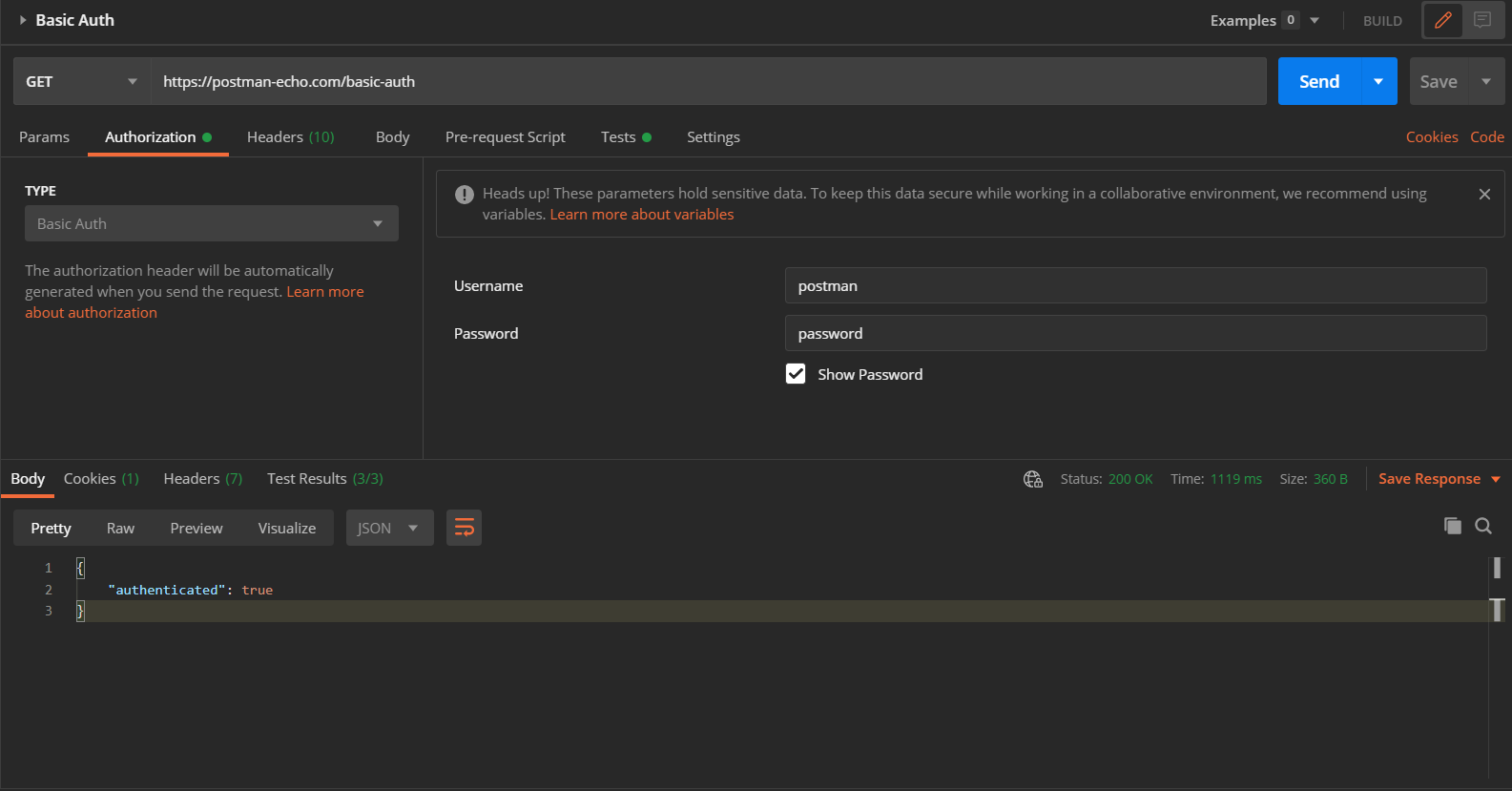
While keeping in mind various points discussed in the earlier section, I have wrote test cases.
pm.test("Check Wether it is Authenticated Request", function () {
var jsonData = pm.response.json();
pm.expect(jsonData.authenticated).to.eql(true);
});
pm.test("Content-Type is present", function () {
pm.response.to.have.header("Content-Type");
});
pm.test("Successful POST request", function () {
pm.response.to.have.status(200);
});
Bearer Token
Bearer authentication (also called token authentication) is an HTTP authentication scheme that involves security tokens called bearer tokens. The name “Bearer authentication” can be understood as “give access to the bearer of this token.”
Here, I am verifying User listing API, and for that I need to have bearer token to bring list of all user and pass token in the API.
How to set bearer token in the postman ?
- Navigate to the Authorization Tab and Select Bearer Token
- Add token
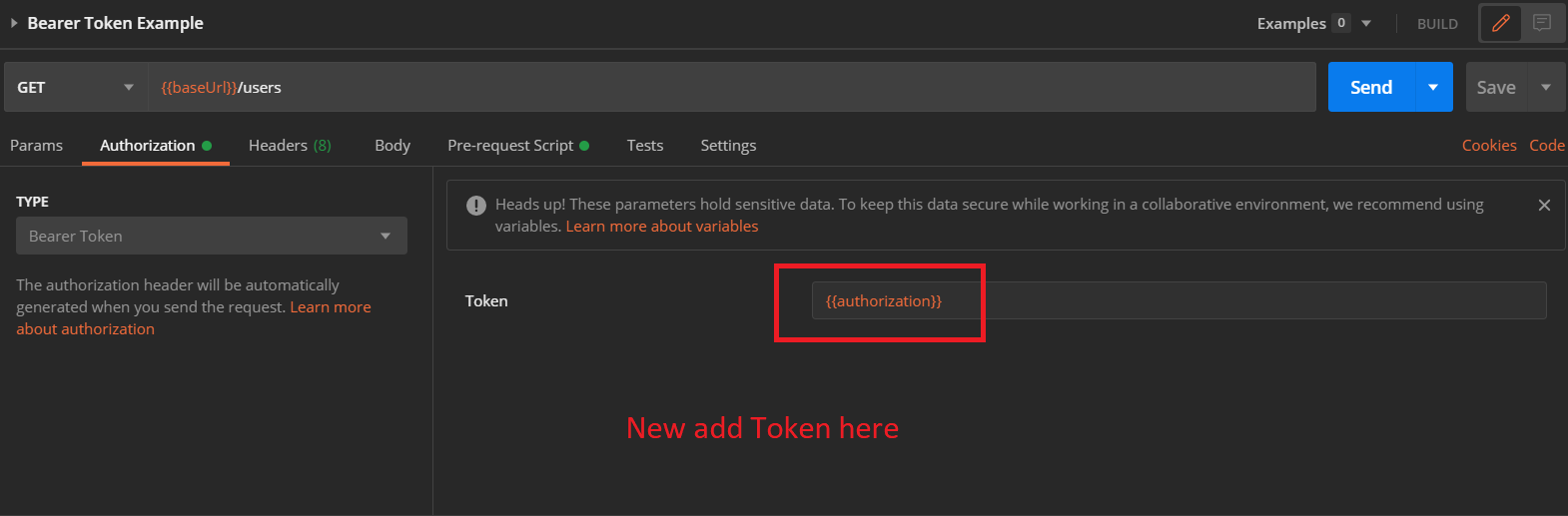
How to set Token in postman env and access ?
- Create a Pre-Request Script for storing token after validating the user.
- `{{authorization}}` accessing the variable in the request from the postman env.
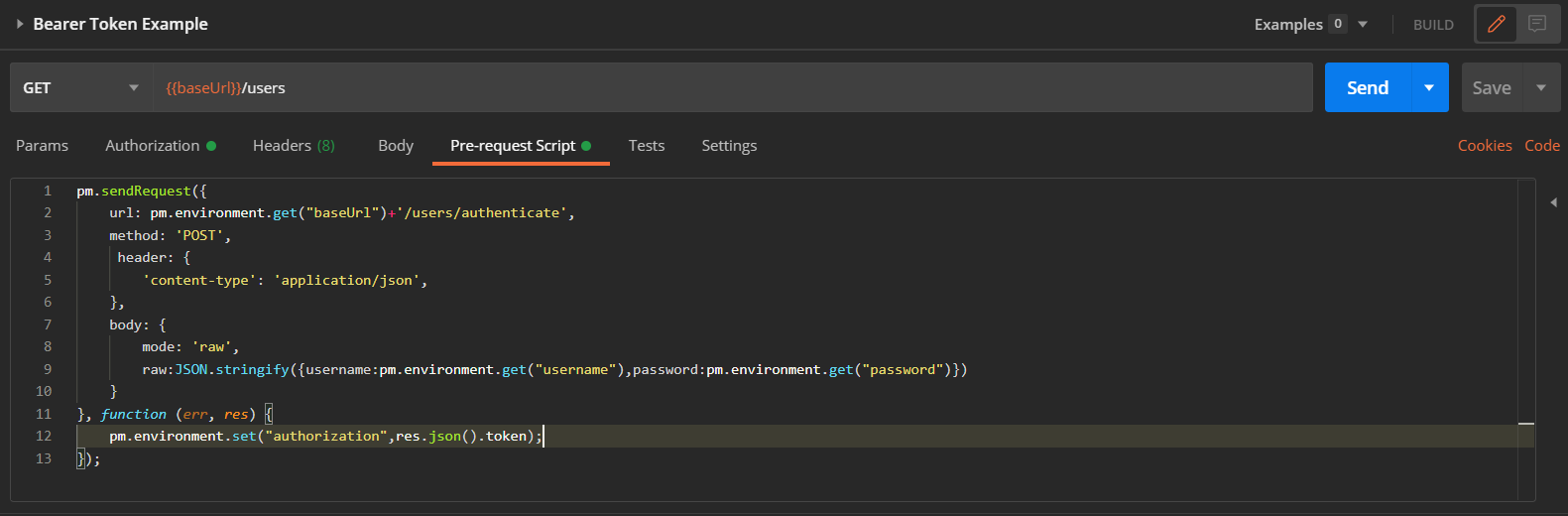
pm.sendRequest({
url: pm.environment.get("baseUrl") + '/users/authenticate',
method: 'POST',
header: {
'content-type': 'application/json',
},
body: {
mode: 'raw',
raw: JSON.stringify({ username: pm.environment.get("username"), password: pm.environment.get("password") })
}
}, function (err, res) {
pm.environment.set("authorization", res.json().token);
});
Let us write Test cases for Getting list of user.
const schema = JSON.parse(environment.listUserSchema);
const jsonData = JSON.parse(responseBody);
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
pm.test("Content-Type is present", function () {
pm.response.to.have.header("Content-Type");
});
pm.test("Check the response is JSON", function () {
pm.response.to.be.json;
pm.response.to.not.be.error;
});
pm.test("Schema Validation",() => {
var result = tv4.validate(jsonData, schema, false, true);
if (result !== true) {
console.log('Schema validation failed:', tv4.error);
console.log(tv4.error.dataPath);
}
pm.expect(result).to.be.true;
});
For schema validation , we need to generate json schema and save to postman env.
For generating json schema you can use this link. In the test we are verify the objects/json are as per schema or not , if there is any modifications in the object/json then this test will fail and will provide detailed log in the postman console.
AWS Signature
Pre-requisites
I will assume that you have an Amazon AWS account and that you are at least a bit familiar with the Amazon Console web interface.
We need to have before hand AWS access key and secret key.
Once you have both access key and secret key we need to store in the postman env. Go to authorization tab and select AWS Signature and postman env keys inside Accesskey and SecertKey and also need to provided Service name of AWS.
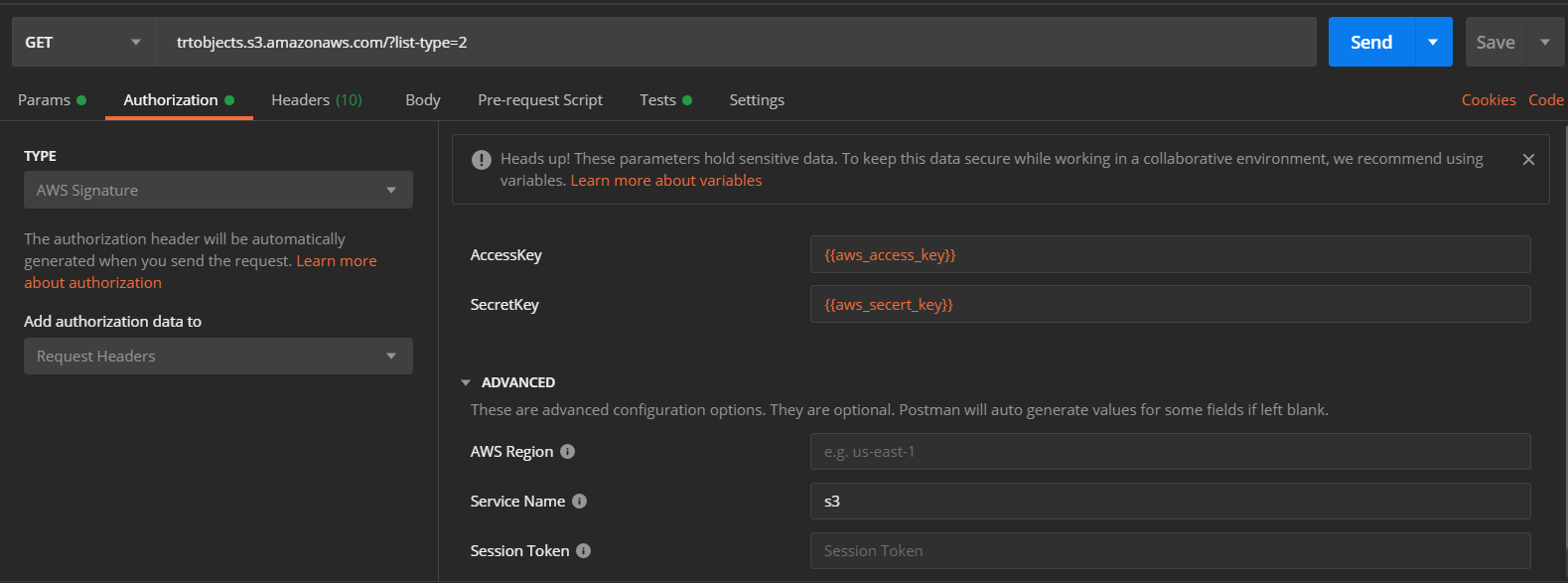
Here, I am demonstrating to get all objects from the S3 to check its header and validate number of objects should be 9
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
pm.test("Check custom headers", function () {
pm.response.to.be.header("Content-Type","application/xml");
pm.response.to.have.header("x-amz-id-2");
pm.response.to.have.header("x-amz-bucket-region");
});
pm.test("Parse response to Json and count the objects", function () {
var jsonObject = xml2Json(responseBody);
pm.response.to.not.be.error;
pm.expect(jsonObject.ListBucketResult.Contents.length).to.be.eq(9)
});
Amazon provides response in XML , so here in the test I am converting XML to JSON so that we can validate the objects given by S3 for the bucket.
OAuth2.0
OAuth 2 is an authorization framework that enables applications to obtain limited access to user accounts on an HTTP service, such as Facebook, GitHub, and many others. It works by delegating user authentication to the service that hosts the user account, and authorizing third-party applications to access the user account.
Here, I am going to demonstrate how we have setup Oauth2.0 authorization in postman what changes we need to set in the postman.
Click on Authorization Tab in postman and select Oauth2.0. and click on Get new Access Token button.
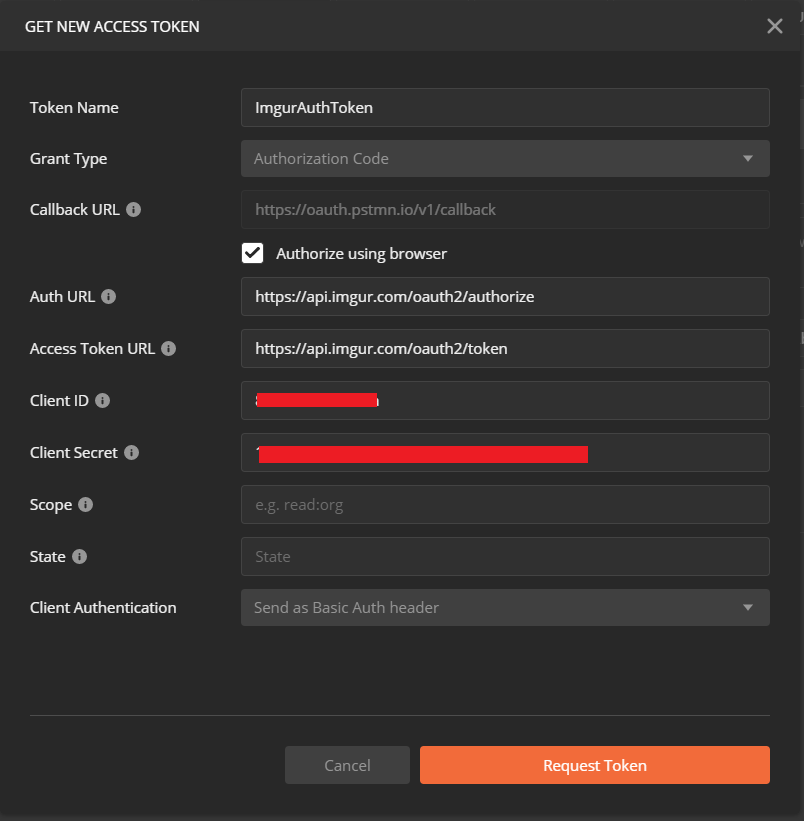
In the above image , we need to fill details regarding what type of Oauth2.0 is used, that we can set by clicking on Grant type. Currently, we need to have Authorization code method. We can change this method as per our requirement.
I am selecting by default Callback URL for Postman itself as I need to test the api in the postman. Generally we need to set callback URL for your application.
In Auth URL , we need to set for endpoint of the authorization of the server from where we can get the authorization code.
Access Token URL , this is used to exchange authorization code from for an access token.
Client ID and Client secret are generated while registration process of the applications.
On Clicking on the Request Token , it will open browser and navigate to application endpoint will get the access token.
Once we get token it will be shown in the Access token field.
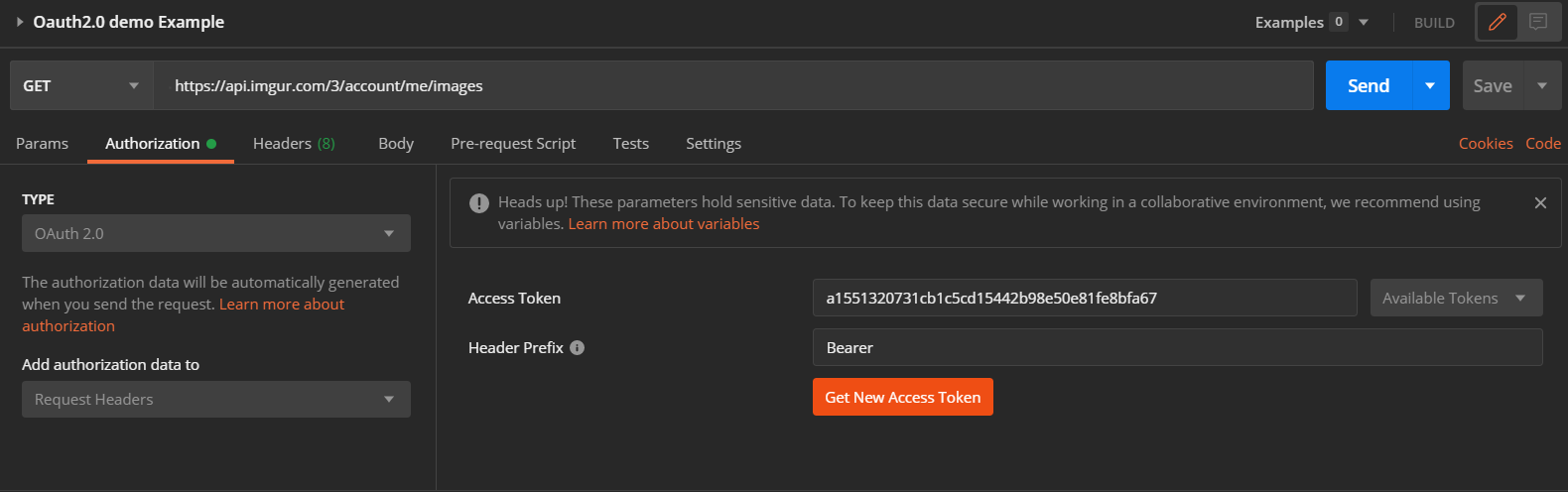
Now we are going to write Test case for getting images from my account and validate that it should have name, which type of image it is and it should have a image link in the object.
const schema = JSON.parse(environment.listImageSchema);
const jsonData = JSON.parse(responseBody);
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
pm.test("Content-Type is present", function () {
pm.response.to.be.header("Content-Type","application/json");
});
pm.test("Check the response is JSON", function () {
pm.response.to.be.json;
pm.response.to.not.be.error;
});
pm.test("Check name of the Image", function () {
pm.expect(jsonData.data[0].name).to.eq('QA')
});
pm.test("Check type and url of the Image", function () {
pm.expect(jsonData.data[0].type).to.eq('image/png')
pm.expect(jsonData.data[0].link).to.eq('https://i.imgur.com/cpF9unR.png')
});
pm.test("Schema Validation",() => {
var result = tv4.validate(jsonData, schema, false, true);
if (result !== true) {
console.log('Schema validation failed:', tv4.error);
console.log(tv4.error.dataPath);
}
pm.expect(result).to.be.true;
});